If you’ve set your sights on a software development career, you’re probably aware of the challenges involved in cracking the coding interview. Whether you’re a newbie or a seasoned professional, acing those technical questions can be nerve-wracking. That’s why we’ve created this comprehensive guide to help you master the art of cracking the coding interview. From understanding algorithms to mastering data structures, we’ll walk you through each step of the process.
Table of Contents
Introduction to Cracking the Coding Interview
In today’s rapidly advancing technological landscape, software development has become one of the most sought-after careers. With opportunities spanning various industries and disciplines, the competition for coveted positions in top tech companies has grown fiercer than ever. That’s why cracking the coding interview has become an essential milestone for aspiring programmers.
But what does cracking the coding interview entail, and why is it so pivotal in the journey toward landing your dream job? Let’s explore the key elements:
The Coding Interview Landscape
Coding interviews have evolved from mere discussions about technical skills to rigorous evaluations of a candidate’s ability to think logically, solve problems, and communicate effectively. Cracking the coding interview means showcasing not only your coding abilities but also your understanding of algorithms, data structures, and software design principles.
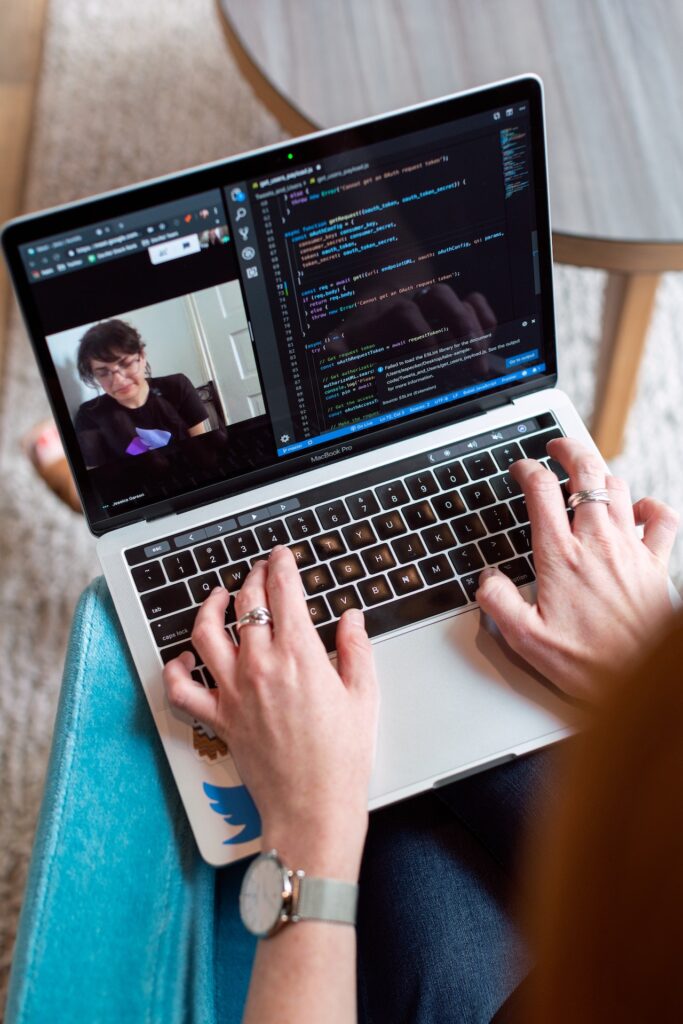
The Challenge of Cracking the Coding Interview
For many, the prospect of a coding interview can be daunting. Questions can range from simple programming tasks to complex algorithmic challenges. The pressure of performing well in a time-constrained environment adds to the anxiety. Cracking the coding interview requires preparation, practice, and a strategic approach.
Why Cracking the Coding Interview Matters
Cracking the coding interview is not just about getting a job; it’s about proving your mettle in a field that demands innovation, creativity, and technical excellence. It’s about demonstrating your ability to adapt, learn, and excel in a constantly changing industry. It’s your ticket to opportunities, growth, and success in the tech world.
How This Guide Can Help
This comprehensive guide is designed to equip you with the knowledge, tools, and confidence needed to crack the coding interview. Whether you’re just starting your career or looking to take the next big leap, our step-by-step approach will help you navigate the complexities of coding interviews. From understanding core concepts to mastering the art of problem-solving, we’ll be with you every step of the way.
Cracking the coding interview is no small feat, but it’s far from insurmountable. With the right mindset, preparation, and guidance, you can turn this challenge into a triumph. This guide is your roadmap, filled with insights, strategies, and support to help you achieve your career aspirations.
The Importance of Algorithm Knowledge
The role of algorithms in software development is undeniable, but when it comes to cracking the coding interview, the importance of algorithm knowledge is magnified exponentially. In this section, we’ll explore why understanding algorithms is so crucial and how it can be the defining factor in your success.
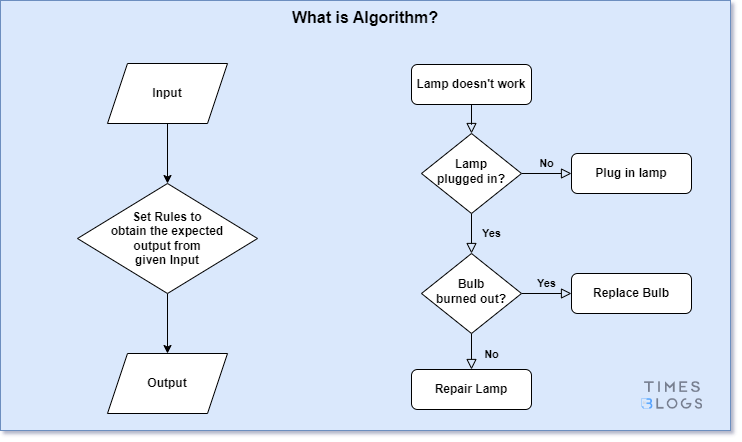
Algorithms: The Heart of Problem-Solving
In computer science, algorithms are step-by-step procedures for solving a problem or accomplishing a task. They are the building blocks of efficient programming, allowing developers to create solutions that are not only correct but also optimized in terms of time and space complexity.
Why Focus on Algorithms for Cracking the Coding Interview?
- Assessment of Analytical Skills: Coding interviews often include algorithmic challenges to assess a candidate’s ability to think analytically and approach problems systematically. Understanding algorithms helps in breaking down complex problems into manageable parts.
- Efficiency Matters: Cracking the coding interview isn’t just about finding a solution; it’s about finding the best solution. Knowledge of algorithms helps in devising efficient solutions that make optimal use of resources.
- Versatility Across Languages: Algorithms are fundamental concepts that apply across programming languages. Whether you code in Python, Java, or C++, the underlying algorithmic principles remain the same. Mastering algorithms prepares you for interviews, regardless of the language requirements.
- Real-World Applications: Understanding algorithms is not just for cracking the coding interview; it’s a skill that translates into real-world software development. From search engines to social media platforms, algorithms power the technology we use every day.
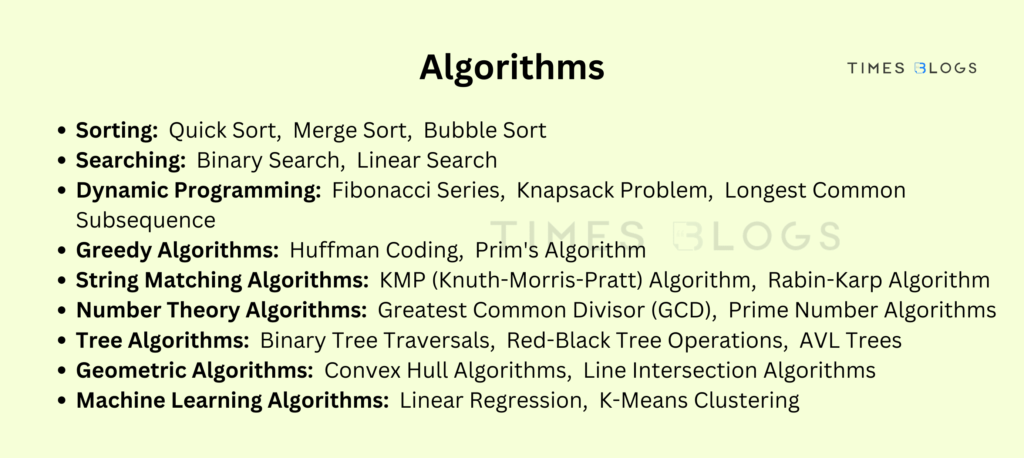
Key Algorithms to Know
- Sorting Algorithms
- Quick Sort: Efficient for large datasets.
- Merge Sort: Uses a divide-and-conquer approach.
- Bubble Sort: Easy to understand but less efficient.
- Search Algorithms
- Binary Search: Fast search algorithm for sorted arrays.
- Linear Search: Simple search through each element.
- Graph Algorithms
- Dijkstra’s Algorithm: Finds the shortest path between nodes.
- Floyd-Warshall Algorithm: Solves all pairs of shortest paths.
- Kruskal’s Algorithm: Finds the minimum spanning tree.
- Breadth-First Search (BFS): Explores nodes level by level.
- Depth-First Search (DFS): Explores as far as possible along each branch.
- Dynamic Programming
- Fibonacci Series: Using memoization or bottom-up approach.
- Knapsack Problem: A classic optimization problem.
- Longest Common Subsequence: Finds common subsequences in strings.
- Greedy Algorithms
- Huffman Coding: Used for lossless data compression.
- Prim’s Algorithm: Another way to find the minimum spanning tree.
- String Matching Algorithms
- KMP (Knuth-Morris-Pratt) Algorithm: Efficient string searching.
- Rabin-Karp Algorithm: Utilizes hashing for pattern searching.
- Number Theory Algorithms
- Greatest Common Divisor (GCD): Uses Euclid’s algorithm.
- Prime Number Algorithms: Sieve of Eratosthenes for finding primes.
- Tree Algorithms
- Binary Tree Traversals: In-order, pre-order, post-order traversals.
- Red-Black Tree Operations: Understanding balanced search trees.
- AVL Trees: Another form of a self-balancing binary search tree.
- Geometric Algorithms
- Convex Hull Algorithms: Such as Graham’s scan.
- Line Intersection Algorithms: Finding intersections of lines.
- Machine Learning Algorithms (Optional)
- Linear Regression: Basic predictive modeling.
- K-Means Clustering: Unsupervised learning for clustering.
Understanding these algorithms not only equips you with the necessary tools for solving a wide range of problems but also showcases your depth of knowledge in computer science. By mastering these, you’re taking significant strides toward cracking the coding interview.
Cracking the coding interview requires more than just coding skills; it demands a deep and nuanced understanding of algorithms. By studying algorithms, you’re not only preparing for interviews but also investing in a skill that will serve you throughout your career.
Whether you’re a beginner aiming to understand the basics or an experienced developer seeking to sharpen your knowledge, focusing on algorithms will set you on the path to success. Remember, cracking the coding interview is not just about getting the job; it’s about proving your prowess in a field that thrives on problem-solving, efficiency, and innovation.
Understanding Data Structures: A Key to Cracking the Coding Interview
When it comes to cracking the coding interview, understanding data structures is not just an advantage; it’s a necessity. Data structures are foundational elements of programming that allow us to organize, store, and manage data efficiently. In this section, we’ll explore the essential data structures, their importance in coding interviews, and how they fuel the solutions to complex problems.
What Are Data Structures?
Data structures are specific ways of organizing and storing data so that it can be accessed and modified efficiently. They play a vital role in various computing applications and form the core of computer science.
Why Data Structures Are Crucial for Cracking the Coding Interview
- Efficiency in Problem-Solving: Different problems require different solutions. Selecting the appropriate data structure can be the key to creating an efficient solution, thereby aiding in cracking the coding interview.
- Versatility in Coding: Understanding various data structures enables you to approach problems from different angles, showcasing your adaptability and creativity in problem-solving.
- Demonstration of Deep Knowledge: Interviews often probe your grasp of core computer science concepts. A thorough understanding of data structures exhibits your foundational knowledge and dedication to the craft.
- Real-World Applicability: Just like algorithms, data structures are not only important for interviews but are also crucial in everyday coding practices. They help in building scalable, efficient, and maintainable code.
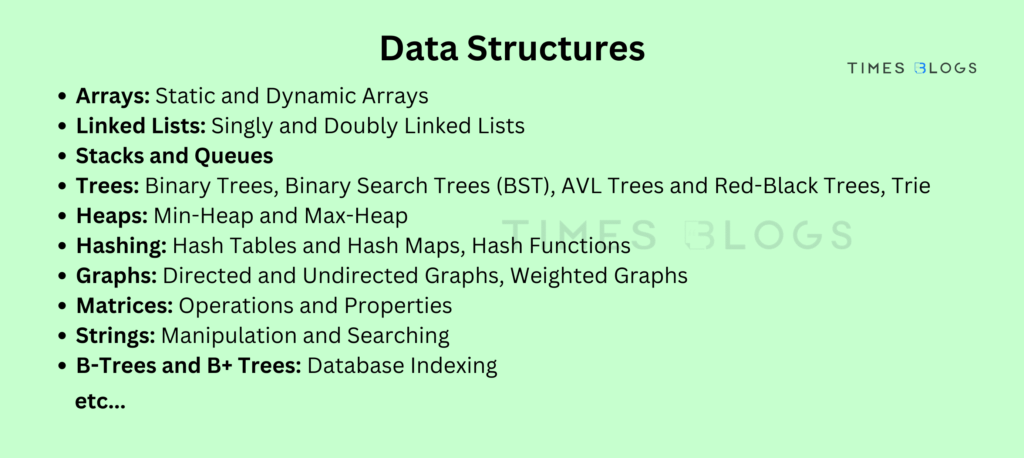
Essential Data Structures to Master
- Arrays
- Static and Dynamic Arrays: Knowing the difference and when to use each type.
- Linked Lists
- Singly and Doubly Linked Lists: Understanding traversal, insertion, and deletion.
- Stacks and Queues
- Implementation and Usage: Learning how to use these in different scenarios, such as parsing expressions.
- Trees
- Binary Trees: Includes understanding various traversals.
- Binary Search Trees (BST): Operations and balancing techniques.
- AVL Trees and Red-Black Trees: Self-balancing trees.
- Trie: Used in text processing and searching algorithms.
- Heaps
- Min-Heap and Max-Heap: Utilized in algorithms like heap sort and priority queues.
- Hashing
- Hash Tables and Hash Maps: Understanding collision resolution techniques.
- Hash Functions: Crafting and recognizing good hash functions.
- Graphs
- Directed and Undirected Graphs: Understanding adjacency lists and matrices.
- Weighted Graphs: Applicable in network flow and shortest path problems.
- Matrices
- Operations and Properties: Including multiplication, inversion, and transposition.
- Strings
- Manipulation and Searching: Including algorithms like Rabin-Karp.
- B-Trees and B+ Trees
- Database Indexing: Utilized in databases for efficient searching and indexing.
- Suffix Trees and Arrays
- Advanced String Processing: Used in algorithms involving string matching and manipulation.
- Bloom Filters
- Probabilistic Data Structure: For testing whether an element is a member of a set.
- Disjoint-Set Data Structures (Union-Find)
- Set Operations: Understanding union and find operations in disjoint sets.
- Segment Trees and Fenwick Trees
- Range Queries: Useful for range queries and updates in arrays.
- Spatial Data Structures
- Quadtree, Octree, and KD-Trees: Used in computer graphics and spatial partitioning.
- Probabilistic Data Structures
- Count-Min Sketch, HyperLogLog: For approximate frequency counting and cardinality estimation.
Understanding data structures is a fundamental skill that transcends coding interviews and shapes the way we think as programmers. By mastering these building blocks, you’re not only increasing your chances of cracking the coding interview but also equipping yourself with tools that will benefit your entire programming career.
Common Coding Interview Questions: Preparing for Success
One of the most practical ways to prepare for success and move closer to cracking the coding interview is to familiarize yourself with common coding interview questions. By understanding what to expect, you can approach your interview with confidence and agility. In this section, we’ll explore some typical questions that reflect the areas we’ve previously discussed, including algorithms and data structures.
1. Array and String Manipulation
- Question: “Reverse an array without using additional memory.”
- Question: “Check if a string is a palindrome.”
These questions test your ability to manipulate basic data structures and understand their properties.
2. Linked Lists
- Question: “Find the middle element of a linked list.”
- Question: “Detect a cycle in a linked list.”
Understanding the dynamics of linked lists is often assessed in interviews, as they form the basis of many complex structures.
3. Trees and Graphs
- Question: “Implement a binary search tree.”
- Question: “Find the shortest path between two nodes in an unweighted graph.”
These questions evaluate your grasp of hierarchical structures and traversal techniques.
4. Sorting and Searching
- Question: “Implement the quicksort algorithm.”
- Question: “Search for an element in a rotated sorted array.”
Your ability to sort and search data efficiently can be vital in cracking the coding interview.
5. Dynamic Programming
- Question: “Solve the knapsack problem.”
- Question: “Find the nth Fibonacci number using dynamic programming.”
These questions demonstrate your problem-solving skills and understanding of optimization techniques.
6. System Design
- Question: “Design a URL shortening service like Bitly.”
- Question: “Explain how you would design a cache system.”
System design questions assess your ability to think broadly and architect complex systems.
7. Hash Tables
- Question: “Find the first non-repeated character in a string.”
- Question: “Implement a hashmap from scratch.”
These questions gauge your understanding of hashing mechanisms and efficient data retrieval.
8. Stacks and Queues
- Question: “Implement a queue using two stacks.”
- Question: “Write a function to sort a stack.”
These questions assess your grasp of LIFO and FIFO principles and your ability to creatively use these structures.
9. Recursion
- Question: “Write a recursive function to compute the factorial of a number.”
- Question: “Implement the Tower of Hanoi algorithm using recursion.”
Recursion questions test your ability to think in a self-referential way, which is fundamental in algorithm design.
10. Databases and SQL
- Question: “Write a SQL query to find the top 3 highest-paying customers.”
- Question: “Design a database schema for an e-commerce website.”
These questions evaluate your understanding of database management, SQL queries, and how data is stored and retrieved in a relational database.
11. Concurrency and Multithreading
- Question: “Explain how you would implement a thread-safe singleton.”
- Question: “Write a program that prints numbers sequentially using three threads.”
Questions about concurrency and multithreading assess your ability to manage simultaneous processes, a crucial skill in modern software development.
12. Networking and APIs
- Question: “Describe how DNS lookup works.”
- Question: “How would you design a rate-limiter for an API?”
These questions touch on networking principles and how systems communicate with each other, reflecting real-world development scenarios.
Cracking the coding interview is no small feat, and preparation is key. By understanding and practicing common interview questions, you can step into your interview with clarity and self-assurance.
Remember, these questions are not just about finding the right answers; they’re about demonstrating your thinking process, your approach to problem-solving, and your ability to communicate your thoughts effectively.
Invest time in studying these patterns, practice coding them, and learn to explain your reasoning. With dedication and focus, you’ll not only be ready to face these common questions but also equipped to tackle unexpected challenges.
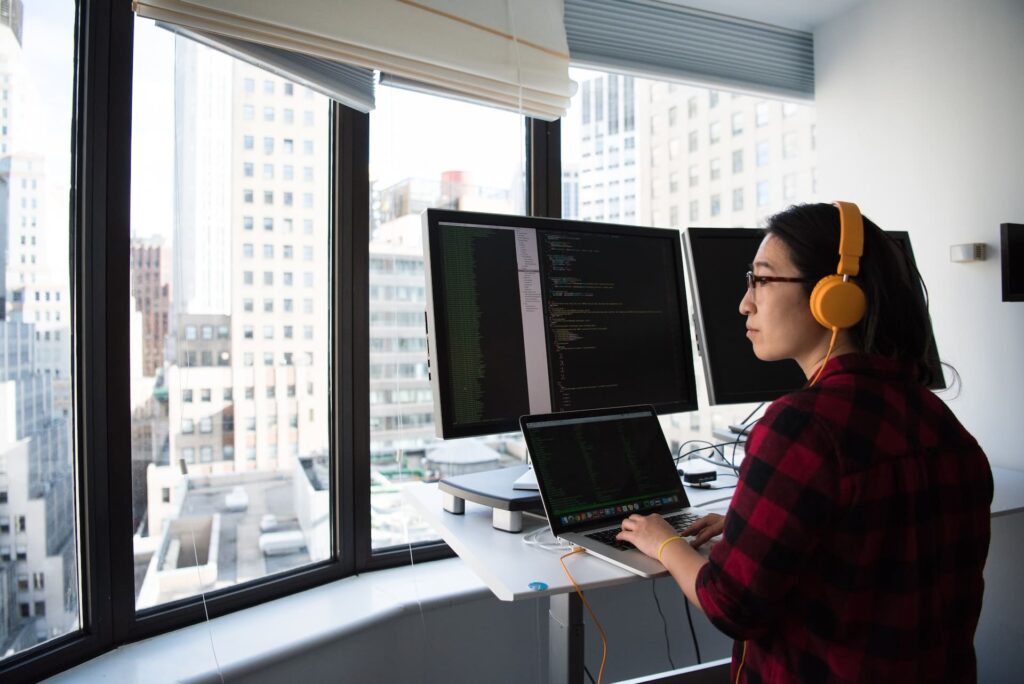
Cracking the Coding Interview: Practice Makes Perfect
The path to cracking the coding interview is filled with challenges, uncertainties, and opportunities for growth. While understanding algorithms, mastering data structures, and familiarizing oneself with common interview questions are crucial, there’s one element that binds all these together: practice. In this section, we’ll explore why practice is the linchpin of success and how you can create a practice routine that will set you on the path to cracking the coding interview.
Why Practice Is Essential?
- Building Confidence: The more you practice, the more familiar you become with problem-solving patterns and coding techniques. This familiarity breeds confidence, allowing you to approach interviews with poise and assurance.
- Honing Skills: Cracking the coding interview requires a blend of theoretical knowledge and practical ability. Regular practice helps you translate what you’ve learned into actionable skills, sharpening your coding prowess.
- Improving Speed and Accuracy: Interviews are time-bound, and efficiency matters. Practice helps you develop the ability to code accurately under time constraints, a key factor in cracking the coding interview.
- Enhancing Communication: Coding interviews aren’t just about writing code; they’re about explaining your thought process. Practice enables you to articulate your solutions clearly and convincingly, a vital skill in the interview process.
Creating a Practice Routine
- Start with Basics: Focus on fundamental problems first. Build a strong foundation in arrays, strings, recursion, and other core concepts before moving to more complex challenges.
- Embrace Variety: Ensure that your practice covers a wide array of topics, from data structures and algorithms to system design and databases.
- Time Yourself: Simulate the interview environment by setting time limits for solving problems. This will help you gauge your speed and adapt to time pressures.
- Learn from Mistakes: Analyze your solutions, identify areas for improvement, and iterate. Learning from mistakes and refining your approach is key to growth.
- Join Coding Platforms: Platforms like LeetCode, HackerRank, and Codewars offer a plethora of problems to solve, ranging in difficulty and topic. Engaging with these communities can be highly beneficial.
- Consider Mock Interviews: Services like Pramp or practicing with a friend can provide valuable interview simulations, complete with feedback and insights from peers or experienced professionals.
Cracking the coding interview is not a one-time event but a journey of continuous learning, growth, and perseverance. Embracing a rigorous practice routine is your ticket to transforming knowledge into ability, and potential into performance.
Remember, there’s no shortcut to success. Dedication, hard work, and consistent practice are the keys to unlocking the doors of opportunity and cracking the coding interview.
Tips and Techniques for Success: A Strategic Approach to Cracking the Coding Interview
Cracking the coding interview is a feat that requires more than just coding acumen. It calls for a strategic approach, blending technical expertise with soft skills, preparation, and the right mindset. Here are some valuable tips and techniques that can pave the way for success in your coding interviews.
1. Understand the Interview Process
- Research the Company: Different companies have unique interview formats. Familiarize yourself with the specific process of the company you’re interviewing with.
- Know What to Expect: From technical questions to behavioral interviews, understanding what’s expected helps you prepare accordingly.
2. Sharpen Your Technical Skills
- Code by Hand: Practice coding on paper or a whiteboard. It may be part of the interview, and it requires different skills than coding on a computer.
- Focus on Fundamentals: Master the core concepts, including data structures, algorithms, and object-oriented programming.
3. Prepare for Behavioral Questions
- Showcase Soft Skills: Communication, teamwork, and problem-solving are often assessed through behavioral questions. Prepare examples that highlight these skills.
- Align with Company Values: Understand the company’s culture and values, and reflect them in your answers.
4. Utilize the STAR Method
- Situation, Task, Action, Result: This method helps structure answers to behavioral questions, providing a coherent and compelling narrative.
5. Practice Problem-Solving, Not Just Coding
- Think Aloud: Explain your thought process as you work through problems. It shows how you approach challenges and can be more important than getting the correct answer.
- Ask Clarifying Questions: If you’re unsure about a problem, ask questions. It demonstrates engagement and critical thinking.
6. Take Care of Logistics
- Test Your Tech: If it’s a virtual interview, ensure your technology works smoothly.
- Dress Appropriately: Professional attire, even for virtual interviews, creates a positive impression.
7. Cultivate a Growth Mindset
- Learn from Each Experience: Whether successful or not, every interview is a learning opportunity. Reflect on what went well and what you can improve.
- Stay Positive and Resilient: Rejections happen; don’t let them deter you. Stay focused on your goal, and keep improving.
8. Follow the Industry Trends
- Stay Updated: Keep an eye on the latest technology trends, frameworks, and tools. Being knowledgeable about the industry showcases your passion and dedication.
- Participate in Communities: Engage with coding communities, forums, and social media groups. Networking and learning from others can be invaluable.
9. Prepare a Portfolio
- Showcase Your Work: Have a portfolio ready with examples of your projects, contributions to open-source, and any relevant achievements. It adds credibility to your skills.
- Explain Your Role: Be ready to articulate your specific contributions and the technologies used in each project.
10. Mock Interviews and Peer Reviews
- Simulate Real Interviews: Practice with friends, mentors, or through platforms that offer mock interviews. Realistic practice helps you overcome nerves and refine your performance.
- Seek Feedback: Constructive criticism from peers and mentors helps you identify areas for improvement.
11. Mind Your Body Language
- Non-Verbal Communication: Whether in person or through a camera, your body language sends messages. Maintain eye contact, sit up straight, and use gestures that convey confidence.
12. Utilize Online Resources and Books
- Leverage Learning Platforms: Websites, video tutorials, and books like “Cracking the Coding Interview” by Gayle Laakmann McDowell provide targeted preparation material.
- Join Coding Challenges: Participate in online coding competitions and hackathons. They offer fun and engaging ways to improve your skills.
13. Take Care of Your Well-being
- Sleep and Nutrition: Ensure you are well-rested and nourished before the interview. Your physical well-being directly impacts your mental performance.
- Manage Stress: Utilize techniques such as meditation or deep breathing to calm nerves. A clear and relaxed mind performs better.
Cracking the coding interview requires a multifaceted strategy, encompassing technical mastery, interpersonal skills, and a proactive and positive attitude. By embracing these tips and techniques, you’re not just preparing for an interview; you’re setting the stage for a thriving career in software development.
Common Mistakes to Avoid: Sidestepping Pitfalls in Cracking the Coding Interview
When embarking on the journey of cracking the coding interview, even the most diligent of candidates may fall into certain traps. Recognizing and avoiding these common mistakes can be as crucial as mastering algorithms and data structures. Here are some frequent missteps and how to evade them:
- Ignoring the Basics
- Mistake: Over-focusing on advanced topics and neglecting fundamental concepts.
- Solution: Build a strong foundation in core programming, algorithms, and data structures.
- Poor Time Management
- Mistake: Spending too much or too little time on certain questions during practice or the actual interview.
- Solution: Practice with time constraints and strategize how to allocate time during the interview.
- Overlooking Behavioral Questions
- Mistake: Focusing exclusively on technical skills and ignoring interpersonal and cultural fit.
- Solution: Prepare for behavioral questions and research the company’s values and culture.
- Not Explaining Thought Processes
- Mistake: Jumping into coding without discussing the approach or thinking aloud.
- Solution: Clearly articulate your thoughts and approach before and during coding.
- Failing to Test Code
- Mistake: Neglecting to run or discuss tests for the written code.
- Solution: Write test cases and explain how the code would handle different scenarios.
- Using Unfamiliar Languages or Tools
- Mistake: Attempting to use a programming language or tool you’re not comfortable with.
- Solution: Stick to what you know best, unless the interviewer specifies otherwise.
- Neglecting Soft Skills
- Mistake: Failing to showcase communication, teamwork, or problem-solving skills.
- Solution: Practice soft skills as part of your interview preparation, such as through mock interviews.
- Ignoring Feedback
- Mistake: Disregarding feedback from peers, mentors, or previous interviews.
- Solution: Actively seek feedback and make necessary improvements.
- Over-Stressing and Burning Out
- Mistake: Overloading yourself with preparation to the point of exhaustion.
- Solution: Plan a balanced preparation schedule, with time for rest and other activities.
- Underestimating the Importance of Practice
- Mistake: Relying solely on theoretical knowledge without hands-on coding practice.
- Solution: Engage in consistent practice through coding platforms, challenges, and personal projects.
Cracking the coding interview requires awareness of potential pitfalls and a proactive approach to avoid them. By recognizing these common mistakes and implementing the corresponding solutions, you place yourself on a path free from unnecessary obstacles.
These insights are not merely cautionary tales but instrumental guidelines that add to your arsenal in cracking the coding interview. Approach them not with fear but with a mindset geared towards continuous learning and improvement.
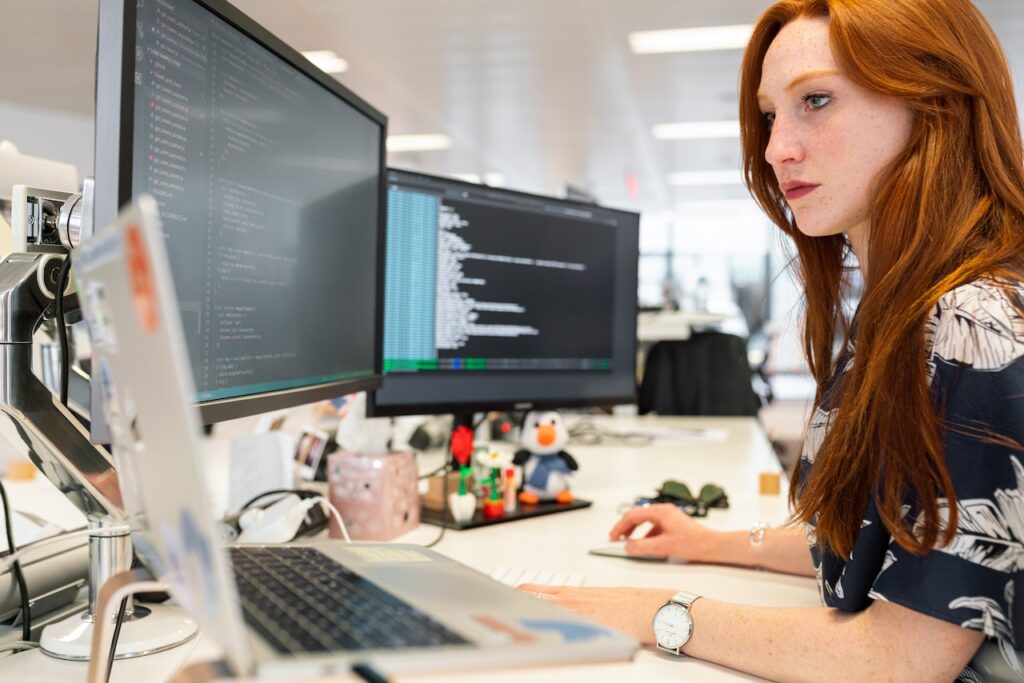
Conclusion: Your Blueprint for Cracking the Coding Interview
Cracking the coding interview is a goal that many aspiring software engineers set their sights on. It’s a multifaceted challenge, requiring not only mastery over technical skills but a combination of strategic preparation, effective communication, problem-solving creativity, and unwavering perseverance.
Through this comprehensive guide, we’ve delved into various dimensions of cracking the coding interview. We’ve explored the importance of algorithms, the nuances of data structures, common coding interview questions, and emphasized how practice makes perfect. We’ve also provided a treasure trove of tips and techniques, covering everything from technical know-how to behavioral insights and personal well-being.
Here’s a quick recap of the crucial takeaways:
- Build Strong Foundations: Focus on algorithms, data structures, and core programming concepts.
- Practice Relentlessly: Simulate interview scenarios, time yourself, and learn from mistakes.
- Embrace a Holistic Approach: Prepare for both technical and behavioral questions, and align with the company’s culture and values.
- Utilize Resources: Leverage books, online platforms, and communities to enrich your preparation.
- Mind the Details: Pay attention to logistics, body language, and your overall well-being.
- Stay Positive and Resilient: Cultivate a growth mindset and stay determined, irrespective of setbacks.
Cracking the coding interview is more than just a pathway to a new job; it’s a journey of self-discovery, skill development, and professional growth. It’s an opportunity to prove to yourself and others what you are truly capable of.
While the road may seem daunting, remember that success is built on a series of small, consistent efforts. Equip yourself with knowledge, surround yourself with motivation, and step forward with confidence. The path to cracking the coding interview is yours to tread, and with this guide in hand, you are well-armed to navigate it successfully.
Your dream job awaits, and the keys to unlock it are now in your possession. Happy coding, and here’s to cracking the coding interview!